Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
subscriber: use ANSI formatting in field formatters (#1702)
Depends on #1696 ## Motivation PR #1696 adds a new API for propagating whether or not ANSI formatting escape codes are enabled to the event formatter and field formatter via the `Writer` type. This means that it's now quite easy to make field formatting also include ANSI formatting escape codes when ANSI formatting is enabled. Currently, `tracing-subscriber`'s default field formatter does not use ANSI formatting --- ANSI escape codes are currently only used for parts of the event log line controlled by the event formatter, not within fields. Adding ANSI colors and formatting in the fields could make the formatted output nicer looking and easier to read. ## Solution This branch adds support for ANSI formatting escapes in the default field formatter, when ANSI formatting is enabled. Now, field names will be italicized, and the `=` delimiter is dimmed. This should make it a little easier to visually scan the field lists, since the names and values are more clearly separated and should be easier to distinguish. Additionally, I cleaned up the code for conditionally using ANSI formatting significantly. Now, the `Writer` type has (crate-private) methods for returning `Style`s for bold, dimmed, and italicized text, when ANSI escapes are enabled, or no-op `Style`s when they are not. This is reused in all the formatter implementations, so it makes sense to have it in one place. I also added dimmed formatting of delimiters in a couple other places in the default event format, which I thought improved readability a bit by bringing the actual *text* to the forefront. Example of the default format with ANSI escapes enabled: 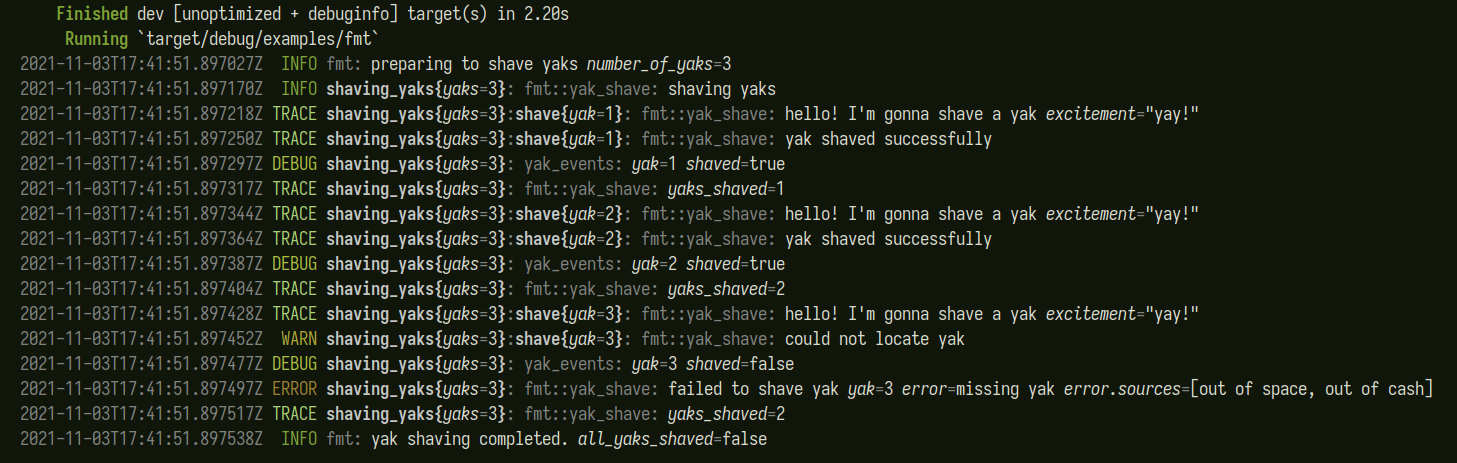 Signed-off-by: Eliza Weisman <eliza@buoyant.io>
- Loading branch information
Showing
2 changed files
with
84 additions
and
41 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters