Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feature #553 Add JSX integration for Vue.js (Kocal)
This PR was squashed before being merged into the master branch (closes #553). Discussion ---------- Add JSX integration for Vue.js Will close #551. Doc: symfony/symfony-docs#11346 This PR enable JSX support in Vue.js with the following code: ```js Encore.enableVueLoader(() => {}, { useJsx: true }); ``` I've added inline documentation and some tests for: - `enableVueLoader()` behavior (and validation) - Babel loader rules generation - Functional test, with styles and scoped styles (using CSS Modules) As proof of concept for styles, after adding `<link href="build/main.css" rel="stylesheet">` in generated `testing.html` file: 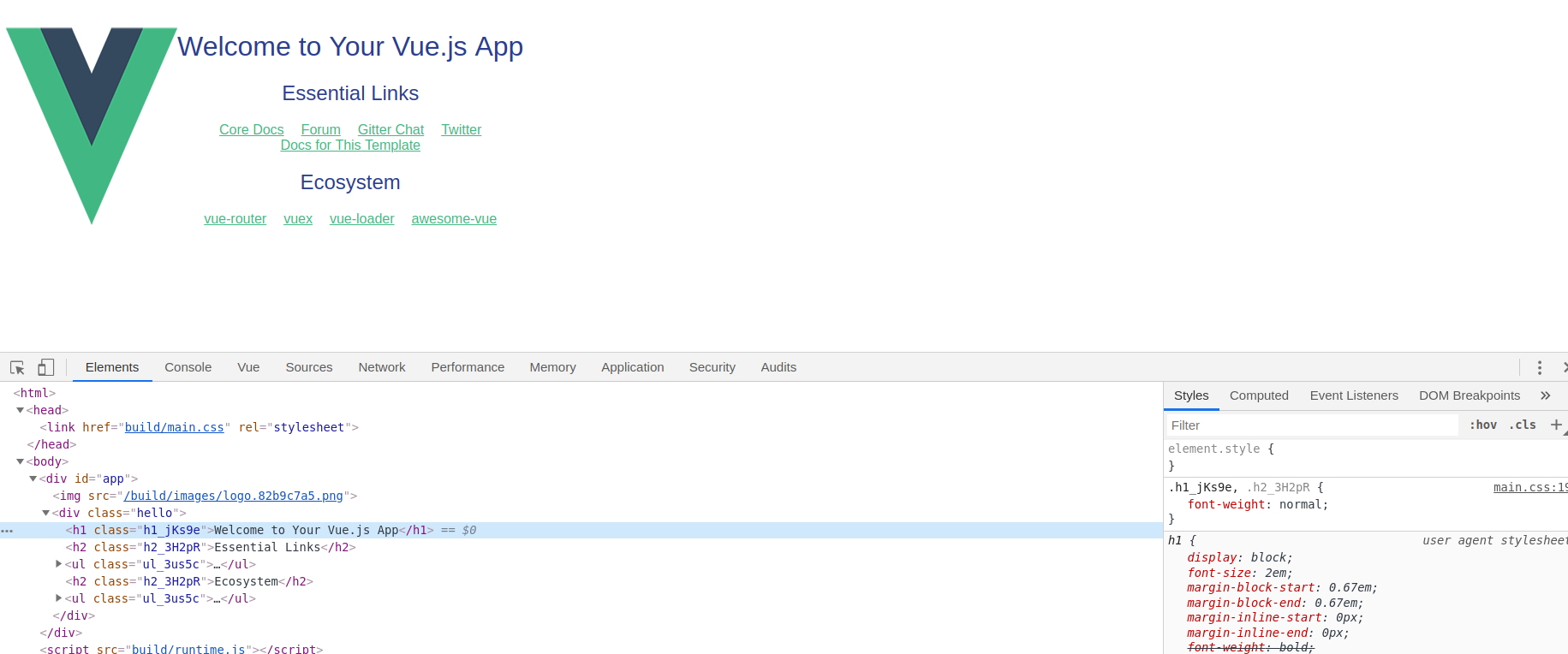 - Styles from `App.css`, `App.scss` and `App.less` are applied globally correctly - Styles from `Hello.css` are applied correctly to `Hello.jsx` component only (`import styles from './Hello.css?module'`) <details> <summary>This is an example of generated `main.css` file</summary> ```css #app { font-family: 'Avenir', Helvetica, Arial, sans-serif; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; text-align: center; color: #2c3e50; margin-top: 60px; } #app { display: flex; color: #2c3e90; } #app { margin-top: 40px; } .h1_jKs9e, .h2_3H2pR { font-weight: normal; } .ul_3us5c { list-style-type: none; padding: 0; } .li_3bINq { display: inline-block; margin: 0 10px; } .a_wKHXy { color: #42b983; } ``` </details> --- Some notes for the documentation: - Install `@vue/babel-preset-jsx` and `@babel/plugin-transform-react-jsx` - If you need to use scoped styles, use [CSS Modules](https://github.com/css-modules/css-modules) like this: ```css /* MyComponent.css */ .title { color: red } ``` ```jsx // MyComponent.jsx import styles from './MyComponent.css'; export default { name: 'MyComponent', render() { return ( <div class={styles.title}> My component! </div> ); } }; ``` - Not only CSS is supported for CSS Modules, Sass, Less and Stylus are supported too - If you need to require an image, `<img src="./assets/image.png">` will not work, you should require it yourself like `<img src={require("./assets.image.png")}/>`. Commits ------- 9cabf9b Add JSX integration for Vue.js
- Loading branch information
Showing
17 changed files
with
338 additions
and
3 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,8 @@ | ||
#app { | ||
font-family: 'Avenir', Helvetica, Arial, sans-serif; | ||
-webkit-font-smoothing: antialiased; | ||
-moz-osx-font-smoothing: grayscale; | ||
text-align: center; | ||
color: #2c3e50; | ||
margin-top: 60px; | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,20 @@ | ||
import './App.css'; | ||
import './App.scss'; | ||
import './App.less'; | ||
import Hello from './components/Hello'; | ||
|
||
class TestClassSyntax { | ||
|
||
} | ||
|
||
export default { | ||
name: 'app', | ||
render() { | ||
return ( | ||
<div id="app"> | ||
<img src={require('./assets/logo.png')}/> | ||
<Hello></Hello> | ||
</div> | ||
); | ||
}, | ||
}; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,3 @@ | ||
#app { | ||
margin-top: 40px; | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,4 @@ | ||
#app { | ||
display: flex; | ||
color: #2c3e90; | ||
} |
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,17 @@ | ||
.h1, .h2 { | ||
font-weight: normal; | ||
} | ||
|
||
.ul { | ||
list-style-type: none; | ||
padding: 0; | ||
} | ||
|
||
.li { | ||
display: inline-block; | ||
margin: 0 10px; | ||
} | ||
|
||
.a { | ||
color: #42b983; | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,33 @@ | ||
import styles from './Hello.css?module'; | ||
|
||
export default { | ||
name: 'hello', | ||
data() { | ||
return { | ||
msg: 'Welcome to Your Vue.js App', | ||
}; | ||
}, | ||
render() { | ||
return ( | ||
<div class="hello"> | ||
<h1 class={styles.h1}>{this.msg}</h1> | ||
<h2 class={styles.h2}>Essential Links</h2> | ||
<ul class={styles.ul}> | ||
<li class={styles.li}><a class={styles.a} href="https://vuejs.org" target="_blank">Core Docs</a></li> | ||
<li class={styles.li}><a class={styles.a} href="https://forum.vuejs.org" target="_blank">Forum</a></li> | ||
<li class={styles.li}><a class={styles.a} href="https://gitter.im/vuejs/vue" target="_blank">Gitter Chat</a></li> | ||
<li class={styles.li}><a class={styles.a} href="https://twitter.com/vuejs" target="_blank">Twitter</a></li> | ||
<br/> | ||
<li class={styles.li}><a class={styles.a} href="http://vuejs-templates.github.io/webpack/" target="_blank">Docs for This Template</a></li> | ||
</ul> | ||
<h2 class={styles.h2}>Ecosystem</h2> | ||
<ul class={styles.ul}> | ||
<li class={styles.li}><a class={styles.a} href="http://router.vuejs.org/" target="_blank">vue-router</a></li> | ||
<li class={styles.li}><a class={styles.a} href="http://vuex.vuejs.org/" target="_blank">vuex</a></li> | ||
<li class={styles.li}><a class={styles.a} href="http://vue-loader.vuejs.org/" target="_blank">vue-loader</a></li> | ||
<li class={styles.li}><a class={styles.a} href="https://github.com/vuejs/awesome-vue" target="_blank">awesome-vue</a></li> | ||
</ul> | ||
</div> | ||
); | ||
}, | ||
}; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,8 @@ | ||
import Vue from 'vue' | ||
import App from './App' | ||
|
||
new Vue({ | ||
el: '#app', | ||
template: '<App/>', | ||
components: { App } | ||
}) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.