-
-
Notifications
You must be signed in to change notification settings - Fork 198
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feature #694 Add Encore.enableBabelTypeScriptPreset() to "compile" Ty…
…peScript with Babel (Kocal) This PR was squashed before being merged into the master branch (closes #694). Discussion ---------- Add Encore.enableBabelTypeScriptPreset() to "compile" TypeScript with Babel See #691, I thought it can be interesting to have a test here. Using Babel to "compile" TypeScript is faster than using `ts-loader` or `tsc` directly, because in fact, it literally remove types annotations. To continue to check types, you have to run `tsc --emitDeclarationOnly` manually (or in a CI). But this is not part of the PR. To migrate an already existing TypeScript app, you just have to configure `babel-loader` to run over `.tsx?` file like this: ```diff Encore - .enableTypeScriptLoader() + .configureLoaderRule('javascript', loader => { + loader.test = /.(j|t)sx?$/; // let Babel to run over .tsx? files too + }) ``` Install some dependencies: `yarn add --dev @babel/preset-typescript @babel/plugin-proposal-class-properties`. And modify your Babel configuration: ```diff { "presets": [ "@babel/env", + "@babel/typescript" ], + "plugins": [ + "@babel/proposal-class-properties" + ] } ``` Maybe I can update `Encore.configureBabel()` and add an option to runs over TypeScript files too... like I did in #574, something like this: ```js Encore .configureBabel(null, { typescript: true }) ``` I've also changed the legacy import/export (`import a = require('...')` to `import a from '...'`). Because it's the legacy way (ES6 imports are very fine) and the Babel TypeScript was not compatible with them: 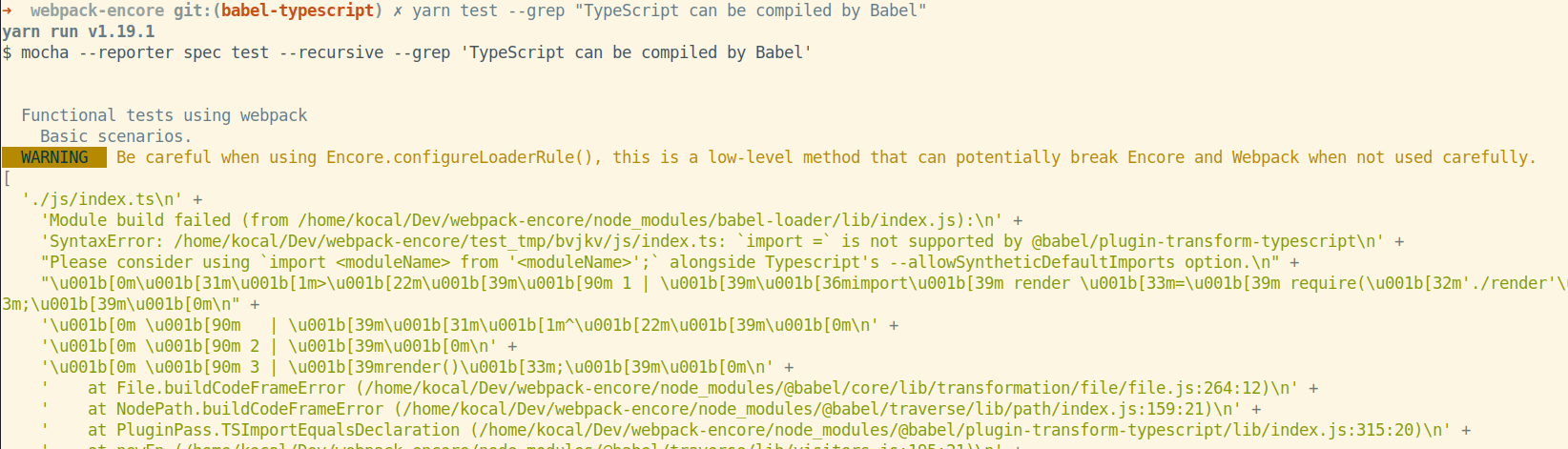 **EDIT :** Added `Encore.enableBabelTypeScriptPreset()` that do all the job for us! :) ```js // simple usage Encore.enableBabelTypeScriptPreset(); // configure TypeScript preset (https://babeljs.io/docs/en/babel-preset-typescript#options) Encore.enableBabelTypeScriptPreset({ isTSX: true; }) ``` `Encore.enableBabelTypeScriptPreset()` can not be used aside `Encore.enableTypeScriptLoader()` or `Encore.enableForkedTypeScriptTypesChecking()`. Commits ------- bdd553a chore: typo 96a666b feat: implement Encore.enableBabelTypeScriptPreset() 6f992b0 feat: prepare method "enableBabelTypeScriptPreset" 8238c32 fixture: add code that only works in TypeScript 66067a0 test: add test for TypeScript "compilation" with Babel 2e21d4f chore(deps-dev): install Babel TypeScript preset, with class properties plugin e053a5e fix(fixtures): use "better" syntax for TypeScript import/export
- Loading branch information
Showing
15 changed files
with
384 additions
and
17 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,3 +1,3 @@ | ||
import render = require('./render'); | ||
import render from './render'; | ||
|
||
render(); | ||
render(); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,5 +1,6 @@ | ||
function render() { | ||
document.getElementById('app').innerHTML = "<h1>Welcome to Your TypeScript App</h1>"; | ||
const html: string = "<h1>Welcome to Your TypeScript App</h1>"; | ||
document.getElementById('app').innerHTML = html; | ||
} | ||
|
||
export = render; | ||
export default render; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,3 +1,5 @@ | ||
{ | ||
"compilerOptions": {} | ||
} | ||
"compilerOptions": { | ||
"allowSyntheticDefaultImports": true | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.