New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
binance: add watchTickers #16159
Merged
Merged
binance: add watchTickers #16159
Changes from all commits
Commits
Show all changes
9 commits
Select commit
Hold shift + click to select a range
ad58afa
binance: add watchTickers
sc0Vu cabe77c
fix not ticker
carlosmiei 2c681d1
Merge branch 'master' of github.com:ccxt/ccxt into add-binance-watch-…
carlosmiei 2d63386
binance: watch bookTickers
sc0Vu bb66488
Merge branch 'add-binance-watch-tickers' of https://github.com/sc0Vu/…
sc0Vu 92b2ab0
binance: update
sc0Vu a9a9fd1
binance: update
sc0Vu cd6a067
fix python transpilation
carlosmiei 18edf5d
Merge branch 'master' of github.com:ccxt/ccxt into add-binance-watch-…
carlosmiei File filter
Filter by extension
Conversations
Failed to load comments.
Jump to
Jump to file
Failed to load files.
Diff view
Diff view
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
@sc0Vu I think we need to apply the same logic as we have in Cex, because, for futures Binance does not send all the updates within the same message, so we end up returning an empty array when the message does not contain the subscribed symbol
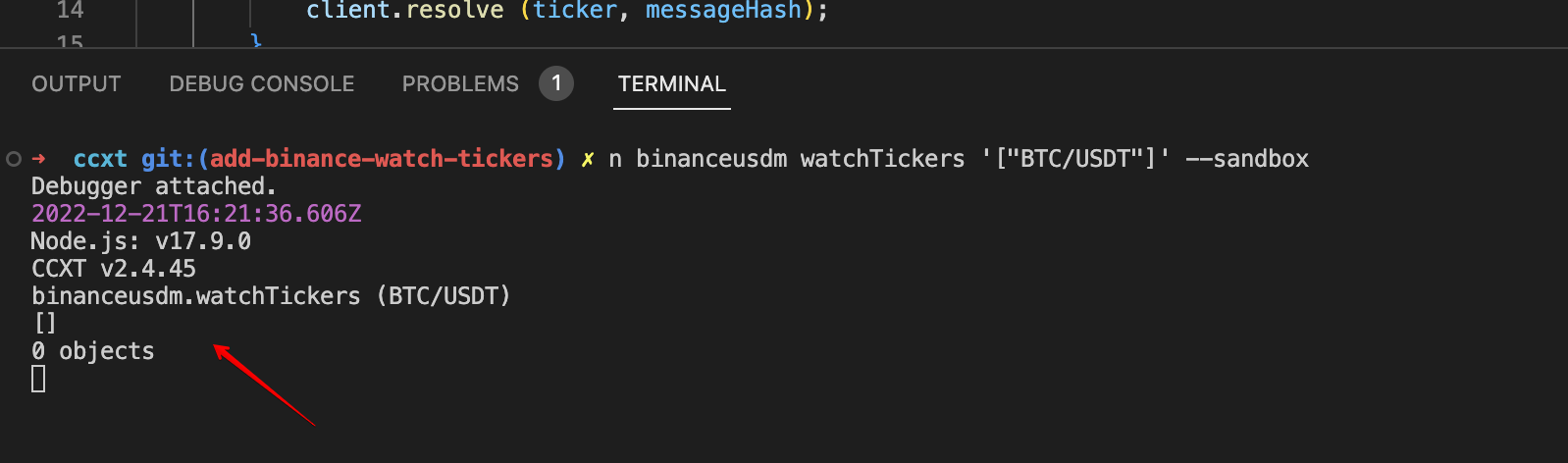
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
It seems the topic and data format are the same for different types, probably need to wait the ticker.
These command worked for me:
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
@sc0Vu what do you mean by "probably need to wait the ticker." ?
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
@carlosmiei I see, you mean user might get empty array in this case.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
@sc0Vu yeah but as I said cex solves this issue in an easy way, can you check it out?
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
@sc0Vu is this ready to be reviewed again?