Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat(cloudwatch): parse all metrics statistics and support long format (
#23095) ### Description Previous PR added support for missing statistics #23074 This PR implements a proper parsing of all these statistics. - Support "short" format `ts99` - Support "long" format - `TS(10%:90%)` | `TS(10:90)` - `TS(:90)` | `TS(:90%)` - `TS(10:)` | `TS(10%:)` - Formats are case insensitive (no breaking changes) - If "long" format and only upper boundary% `TS(:90%)`, can be translated to "short" format `ts90` (`stat.asSingleStatStr`) ### Note I noticed that the following code expected the parsing to throw if it failed, but it actually will **not** fail in any case (it just return `GenericStatistic` if no format matched). I will **not** change this behavior here as I'm not willing to spend more effort testing if this breaks stuff elsewhere. https://github.com/aws/aws-cdk/blob/47943d206c8ff28923e19028acd5991d8e387ac9/packages/%40aws-cdk/aws-cloudwatch/lib/metric.ts#L295-L296 ### Followup work As is, this PR does not change any customer-facing logic. To make use of it, make the parsing throw if no format is recognized. At the end of the parser function, just replace ```ts return { type: 'generic', statistic: stat, } as GenericStatistic; ``` with ```ts throw new UnrecognizedStatisticFormatError() ``` --- You can see all tested inputs here: https://regexr.com/7351s 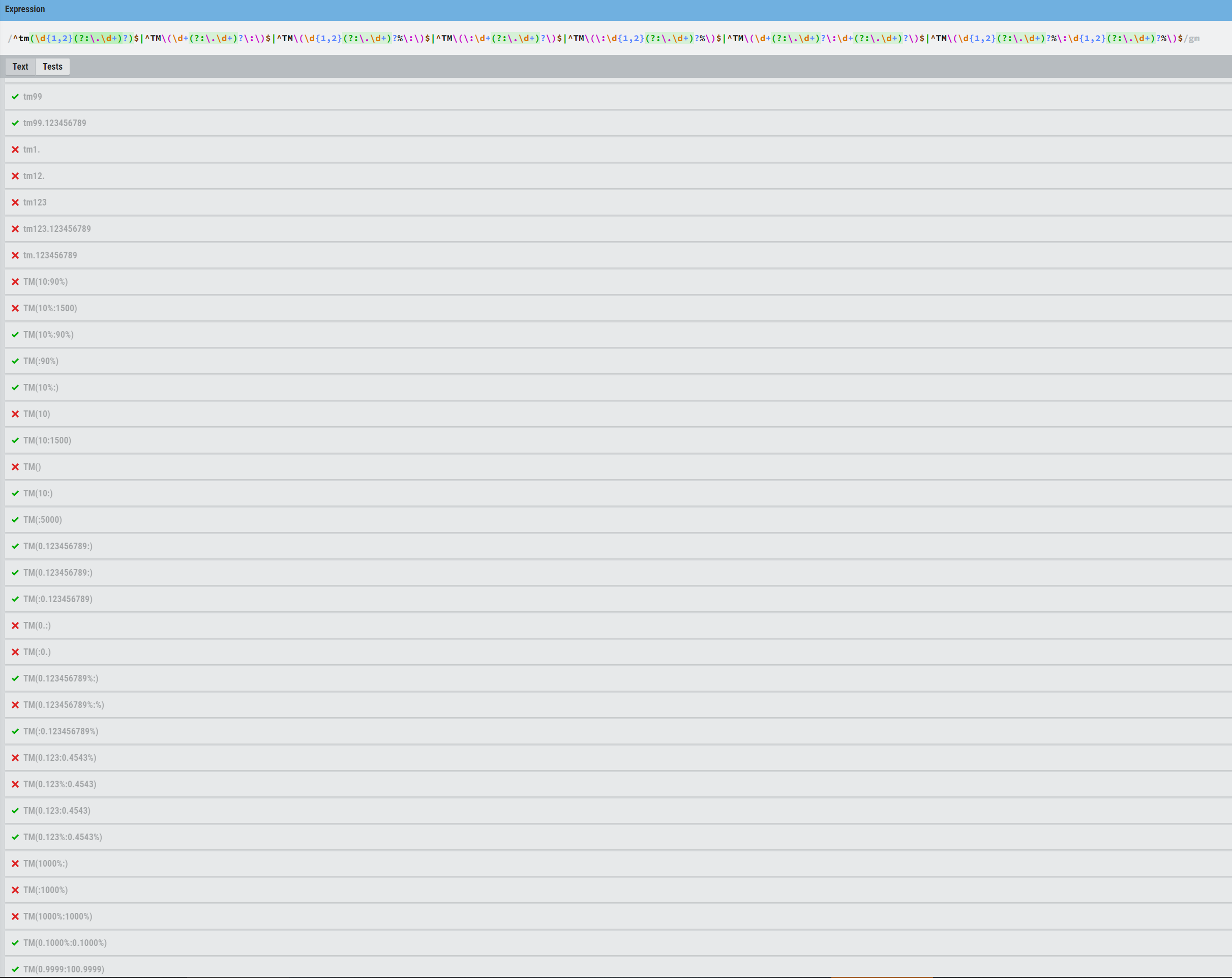 ---- ### All Submissions: * [x] Have you followed the guidelines in our [Contributing guide?](https://github.com/aws/aws-cdk/blob/main/CONTRIBUTING.md) ### Adding new Unconventional Dependencies: * [ ] This PR adds new unconventional dependencies following the process described [here](https://github.com/aws/aws-cdk/blob/main/CONTRIBUTING.md/#adding-new-unconventional-dependencies) ### New Features * [x] Have you added the new feature to an [integration test](https://github.com/aws/aws-cdk/blob/main/INTEGRATION_TESTS.md)? * [ ] Did you use `yarn integ` to deploy the infrastructure and generate the snapshot (i.e. `yarn integ` without `--dry-run`)? *By submitting this pull request, I confirm that my contribution is made under the terms of the Apache-2.0 license*
- Loading branch information
Showing
12 changed files
with
795 additions
and
98 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.