Use plugin along with vue-demi to write Universal Vue Libraries for Vue 2 & 3. #427
Comments
@LinusBorg Thanks for the quick response:
I thought of something similar, but wouldn't this approach build 2 different bundles? (One I do understand that from an architectural level is no easy task tho. |
You will always need two different bundles as soon as components are involved, as Vue 2 and Vue 3 render functions are different. And adding that, there are other breaking changes that will make it difficult to compile the exact same component for Vue 2 and Vue 3, i.e. So thinking about it, compiling components for both Vue 2 and 3 will only be possible for components that use shared APIs exclusively, and/or using additional bridging code that solves these kinds of changes - which might not be possible for all of them. the use case of |
Make sense, thanks for the explanation. |
Created universal Vue2 & Vue3 component with a help of this thread: https://www.npmjs.com/package/@beaubus/single-file-upload-for-vue Here is how I did it: https://www.beaubus.com/blog/make_npm_package_with_vue_single_file_component_sfc_working_with_vue_2_and_3.html |
What problem does this feature solve?
vue-demi is an awesome utility that allows you to write Universal Vue Libraries for Vue 2 & 3.
At the moment, using rollup to build a library, will allow you to externalize Vue demi in the moment of build, similar to what Posva did here in vue-promise:
However, this will only work if the lib you are implementing is only using
typescript
. If the lib requires to have.vue
files for components, as an example, usingrollup-plugin-vue
in the build config will break the library for its use in vue 2 projects.My wild guess is that this rollup plugin uses a specific version of vue because all errors prompt are related to rendering.
Steps to reproduce
git clone https://github.com/alvarosaburido/vue-demi-universal-lib.git
cd examples/vue-2-demo
,yarn
yarn serve
You will get a similar error in the console
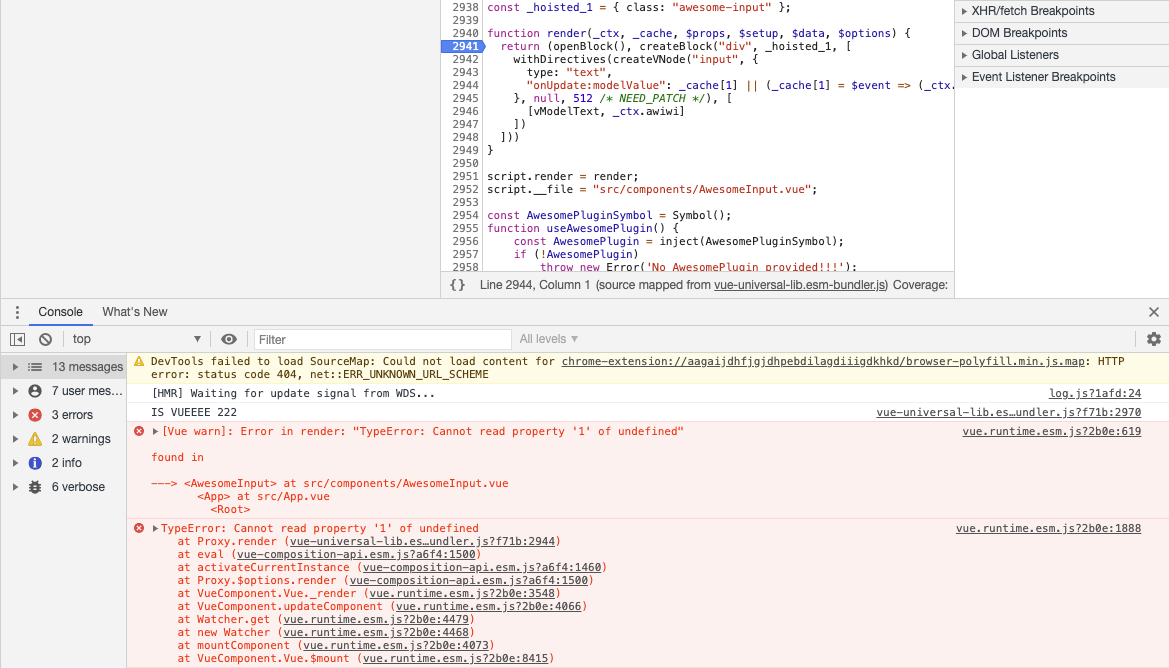
In the
main.js
of thevue-2-demo
(link here) you can toggle between the two versions of the lib:Built with
rollup-plugin-vue
or without it (This version doesn't have
.vue
files, only typescript ones and works correctly)Let me know if it's something that could make sense for this plugin to have. It will open a gate for more universal plugins to avoid maintaining two codebases that double the workload for small scale libraries or new libraries that want to support both versions, doing bugfix or feature supplements twice is just no ideal.
Thanks
What does the proposed API look like?
My rough guess would be to use the
isVue2
property fromvue-demi
to chose between compilers.The text was updated successfully, but these errors were encountered: