New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
feat: @IsOptional should works only for undefined values #491
Comments
I do not understand your problem. I think your test cases are not good. You define property it('should fail on patch when name is undefined', async () => {
const errors = await getValidationErrors({ name: undefined }, PATCH);
expect(errors).not.toEqual([]);
});
it('should fail on patch when name is null', async () => {
const errors = await getValidationErrors({ name: null }, PATCH);
expect(errors).not.toEqual([]);
}); So
|
I use nestjs to validate a DTO from my controller I have this
I send this body json
The expected behaviour:
The actual behaviour:
If I have this DTO
Then
|
I understand now. But as it is a breaking change I want to have more feedback from community. |
I don't know if is the same issue, but i need to validate if a variable is notEmpty, notNull and notUndefined. Is that possible? |
@vlapo How about something like an extra config option? The implementation I've got in my fork doesn't break backwards compatibility (as the option defaults to @isOptional({ nullable: false }) // object.hasOwnProperty(property);
@isOptional({ nullable: true }) // value !== undefined && value !== null
@isOptional() // value !== undefined && value !== null The commit on the fork that adds this behaviour: se-internal@5ac9fa0 |
We would love this as well, ether with a nullable flag, or as a separate set of decorators (although naming them can be difficult). |
Thanks @fhp! Yeah we had originally tried to create a new decorator and must've spent over an hour trying to come up with a good name. Eventually, we decided it made more sense to borrow the design pattern from typeorm's I'll definitely put it into a PR at some point in the next couple of days! Just waiting until I have a moment to add tests. Confirmation from @vlapo or another maintainer that at least the design proposal is accepted would be amazing so I know I'm not doing it for nothing 😄 |
Any update for this? really want to see this feature in next release |
I agree about the strange behavior of this. I like the API proposed by @sam3d. We can use the current behavior as default and add a warning in the changelog about eventually being flipped. I will take a crack at this at the weekend. |
@NoNameProvided Any update? 10 days has been passed |
How about keeping |
/**
* Skips validation if the target is null
*
* @example
* ```typescript
* class TestModel {
* @IsNullable({ always: true })
* big: string | null;
* }
* ```
*/
export function IsNullable(options?: ValidationOptions): PropertyDecorator {
return function IsNullableDecorator(prototype: object, propertyKey: string | symbol): void {
ValidateIf((obj): boolean => null !== obj[propertyKey], options)(prototype, propertyKey);
};
} /**
* Skips validation if the target is undefined
*
* @example
* ```typescript
* class TestModel {
* @IsUndefinable({ always: true })
* big?: string;
* }
* ```
*/
export function IsUndefinable(options?: ValidationOptions): PropertyDecorator {
return function IsUndefinableDecorator(prototype: object, propertyKey: string | symbol): void {
ValidateIf((obj): boolean => undefined !== obj[propertyKey], options)(prototype, propertyKey);
};
} |
For other parts of TypeScript, "optional" means either the property is not set, or it is set to I suggest the following changes to align with the terminology of TypeScript:
|
Meanwhile, I suggest creating two separate decorators: import { ValidateIf } from 'class-validator';
export function CanBeUndefined() {
return ValidateIf((object, value) => value !== undefined);
}
export function CanBeNull() {
return ValidateIf((object, value) => value !== null);
} |
In case anyone is interested, I've had the exact same issue and implemented this (alongside quite a few more decorators) in class-validator-extended, specifically |
This is still an issue, please can this be updated? Some fantastic solutions have been presented. I am happy to make a PR |
any update on this one? And what about |
This code:
gives the following results:
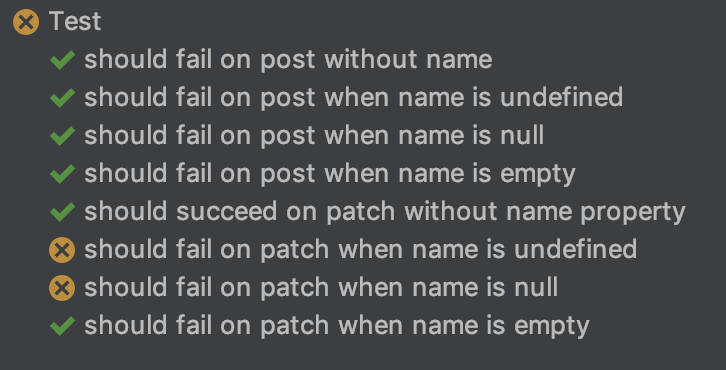
Am I missing something here?
Looking at the code of @IsOptional I see that it checks that the property is not undefined or null, that's why the test for empty string is not failing, but in theory shouldn't it check that this property exists on the object?
The text was updated successfully, but these errors were encountered: