New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
How to accurately obtain the duration of each frame in gif #6887
Labels
Comments
You can get the from PIL import Image, ImageSequence
from PIL import GifImagePlugin
GifImagePlugin.LOADING_STRATEGY = GifImagePlugin.LoadingStrategy.RGB_AFTER_DIFFERENT_PALETTE_ONLY
image_list = []
def test_save_image_list():
im = Image.open(r'old\111111.gif')
fill_color = (255, 255, 255)
duration = []
for frame in ImageSequence.Iterator(im):
duration.append(frame.info["duration"])
frame = frame.convert('RGBA')
bg = Image.new(frame.mode[:-1], frame.size, fill_color)
bg.paste(frame, frame.split()[-1])
frame = bg
# convert_frame = frame.convert('RGB')
# convert_frame.thumbnail((150, 150))
image_list.append(frame)
image_list[0].save(
r'new\111111.gif',
save_all=True,
append_images=image_list[1:],
loop=0,
duration=duration
) |
from PIL import Image, ImageSequence
from PIL import GifImagePlugin
GifImagePlugin.LOADING_STRATEGY = GifImagePlugin.LoadingStrategy.RGB_AFTER_DIFFERENT_PALETTE_ONLY
image_list = []
def test_save_image_list():
im = Image.open(r'old\111111.gif')
fill_color = (255, 255, 255)
for frame in ImageSequence.Iterator(im):
print(f'before: {frame.info}')
frame = frame.convert('RGBA')
bg = Image.new(frame.mode[:-1], frame.size, fill_color)
bg.paste(frame, frame.split()[-1])
frame = bg
# convert_frame = frame.convert('RGB')
# convert_frame.thumbnail((150, 150))
print(f'after: {frame.info}')
image_list.append(frame)
image_list[0].save(
r'\new\111111.gif',
save_all=True,
append_images=image_list[1:],
loop=0
) |
Sure. info_before = frame.info
frame = frame.convert('RGBA')
bg = Image.new(frame.mode[:-1], frame.size, fill_color)
bg.paste(frame, frame.split()[-1])
frame = bg
# convert_frame = frame.convert('RGB')
# convert_frame.thumbnail((150, 150))
frame.info = info_before or if you want just duration, duration_before = frame.info["duration"]
frame = frame.convert('RGBA')
bg = Image.new(frame.mode[:-1], frame.size, fill_color)
bg.paste(frame, frame.split()[-1])
frame = bg
# convert_frame = frame.convert('RGB')
# convert_frame.thumbnail((150, 150))
frame.info["duration"] = duration_before |
Thank you for your answer. My problem has been solved |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
111111.zip
I have a gif composed of multiple pictures. The duration of each picture is different. When I re-compose the gif, I need to know the duration of each picture.
Original gif::
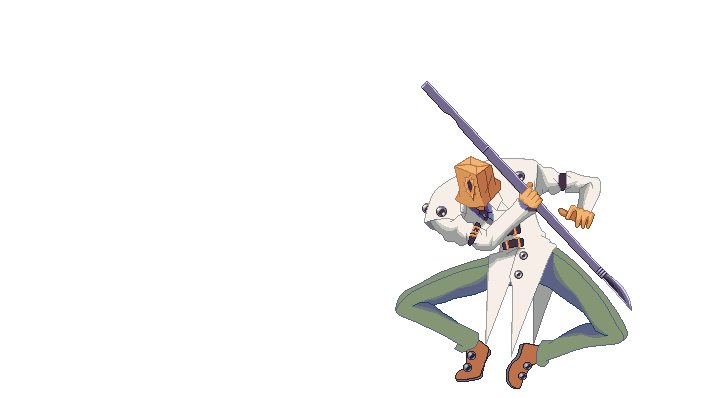
When I use the im object to save, there is no problem with the duration of each image.
Only use im objects to generate new gifs::
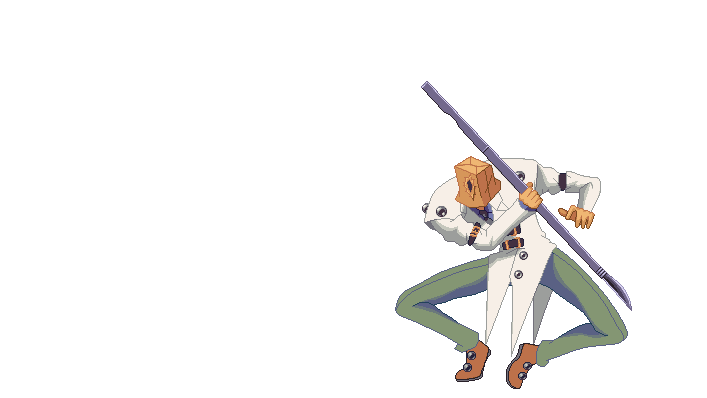
When I split the gif and put each image into a new list, the duration of each image in the resultant gif is also OK.
Gif generated with the new list::
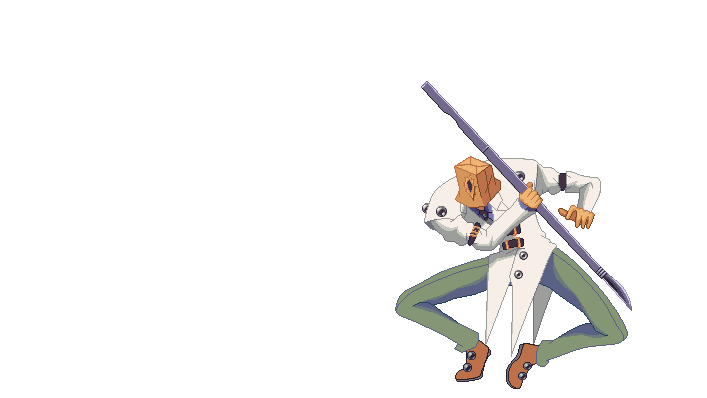
The composite gif also seems to overlap, which may be caused by the transparent background. Let's not discuss this error first.
But when I fill the background or other operations on each image, the duration of each image in the composite gif seems to become the same time. This is different from the duration of each image in the initial gif.In addition, the old gif is in an endless loop (loop=0), and the repetition number of the newly synthesized gif has also changed to 1. I must add another attribute (loop=0) to ensure that it is the result I want.
Gif generated after filling operation::
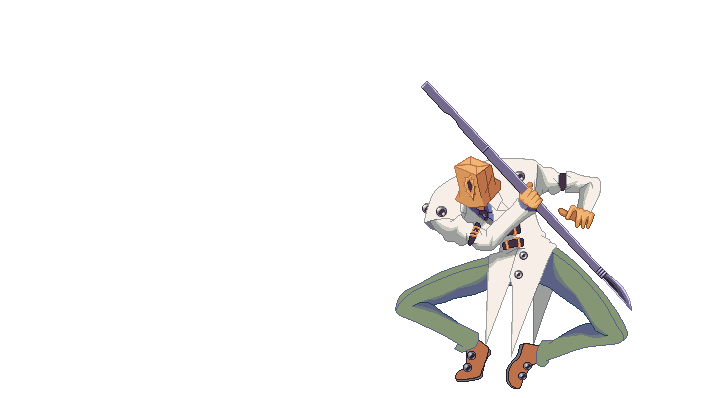
python 3.7.9
Pillow 9.4.0
Before that, I checked the question of #6259 . I think our situation is the same. Therefore, I need to know the correct duration of each frame in the multi-frame picture in the gif, rather than the duration of the first frame.
The text was updated successfully, but these errors were encountered: