You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Short answer:(Style).Faint() does not change the style in some cases.
Long answer: Currently (Style).Faint() uses the faint ANSI sequence. However, this sequence is not properly supported on many terminals as it is often implemented as RGB*2/3. This does not work well on a bright background, as the foreground text is usually close to black in this case and (0, 0, 0) * 2/3 = (0, 0, 0) resulting in no change of color at all or only a barely noticable change. Depending on the color-scheme and implementation the faint text can also end up more pronounced than regular text.
Examples for this would be gnome-terminal or alacritty (to be fair, alacritty allows users to configure an arbitrary color for dim/faint). On the other hand, some terminals such as iTerm or xterm.js implement it by shifting the color towards the background, ensuring a faint or dim appearance regardless of the background color.
Why should this be fixed in termenv?
Because when the users of termenv call (Style).Faint(), they expect text to appear faintly on the screens of the users of their application. As users of termenv, how else would they go about achieving this? They cannot possibly open PRs for each terminal their users may use.
What can we do to fix this?
A color-scheme aware (Style).Faint() implementation could look like the following listing. I found a blendFactor of 0.5 to be in-line of what iTerm does.
Obviously this is a naive implementation that does take into account that the color calculation has to be performed after an t.Foreground(...) calls performed by the user. I'm also not a color expert, so maybe someone will know a better way to blend the colors.
With a slight modification of HasDarkBackground() (see #44), an adaptive faint implemntation that only calculates a blend if the terminal has a light background (which is usually the problematic case):
Alacritty: Alacritty is used an example of the RGB*2/3 faint implementation.
With a light color scheme the current faint rendering produces an even more pronounced color than the regular text. In contrast, the background aware rendering works as expected:
With a dark color scheme the current faint rendering works as intended and the background aware rendering looks the same.
iTerm2: iTerm is used as an example of an color scheme aware faint implementation.
With a light color scheme, the effect of the current faint rendering and the background aware rendering is similar, but the actual color differs slightly.
In the dark color scheme, iTerm2 seems to brighten bold text slightly. The background aware implementation looks a bit different, but that might be due to naive implementation as mentioned above.
Here is some code to test the implementation above:
What's the problem?
Short answer:
(Style).Faint()
does not change the style in some cases.Long answer: Currently
(Style).Faint()
uses the faint ANSI sequence. However, this sequence is not properly supported on many terminals as it is often implemented asRGB*2/3
. This does not work well on a bright background, as the foreground text is usually close to black in this case and(0, 0, 0) * 2/3 = (0, 0, 0)
resulting in no change of color at all or only a barely noticable change. Depending on the color-scheme and implementation the faint text can also end up more pronounced than regular text.Examples for this would be
gnome-terminal
oralacritty
(to be fair, alacritty allows users to configure an arbitrary color for dim/faint). On the other hand, some terminals such asiTerm
orxterm.js
implement it by shifting the color towards the background, ensuring a faint or dim appearance regardless of the background color.Why should this be fixed in
termenv
?Because when the users of
termenv
call(Style).Faint()
, they expect text to appear faintly on the screens of the users of their application. As users oftermenv
, how else would they go about achieving this? They cannot possibly open PRs for each terminal their users may use.What can we do to fix this?
A color-scheme aware
(Style).Faint()
implementation could look like the following listing. I found ablendFactor
of0.5
to be in-line of whatiTerm
does.Obviously this is a naive implementation that does take into account that the color calculation has to be performed after an
t.Foreground(...)
calls performed by the user. I'm also not a color expert, so maybe someone will know a better way to blend the colors.With a slight modification of
HasDarkBackground()
(see #44), an adaptive faint implemntation that only calculates a blend if the terminal has a light background (which is usually the problematic case):Demo
Alacritty: Alacritty is used an example of the
RGB*2/3
faint implementation.With a light color scheme the current faint rendering produces an even more pronounced color than the regular text. In contrast, the background aware rendering works as expected:
With a dark color scheme the current faint rendering works as intended and the background aware rendering looks the same.
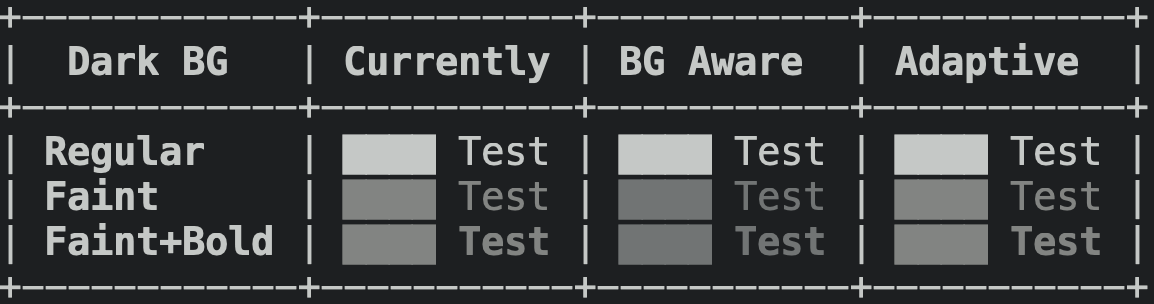
iTerm2: iTerm is used as an example of an color scheme aware faint implementation.
With a light color scheme, the effect of the current faint rendering and the background aware rendering is similar, but the actual color differs slightly.
In the dark color scheme, iTerm2 seems to brighten bold text slightly. The background aware implementation looks a bit different, but that might be due to naive implementation as mentioned above.
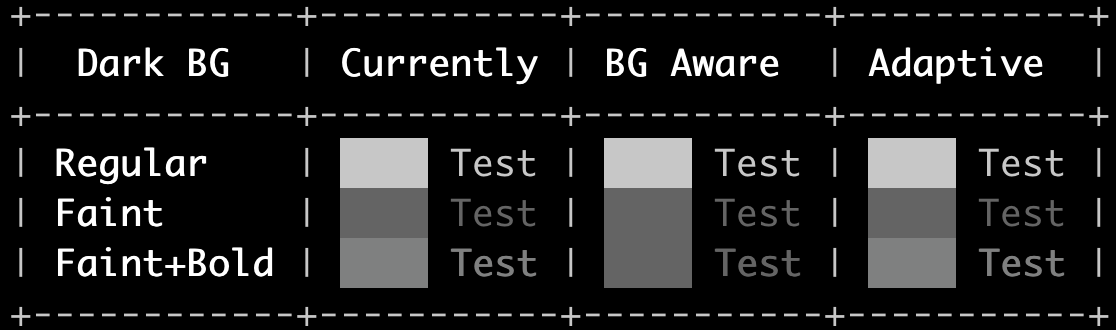
Here is some code to test the implementation above:
Integration
I would suggest adding
(Style).BlendFaint()
and(Style).AdaptiveFaint()
and the respective template functions.If you like this suggestion, I can submit a PR.
The text was updated successfully, but these errors were encountered: